Known Issue
SequenceDiagram Core
1. Export extra large image issue.
To avoid this problem, please increase the java memory setting, or prefer SVG format for export.
It is recommended that you export in SVG format.
Since version 4.0.3, if you choose the JPG, PNG, TIF format, large images will export multiple image tiles, which may be less useful.
SequenceDiagram C/C++
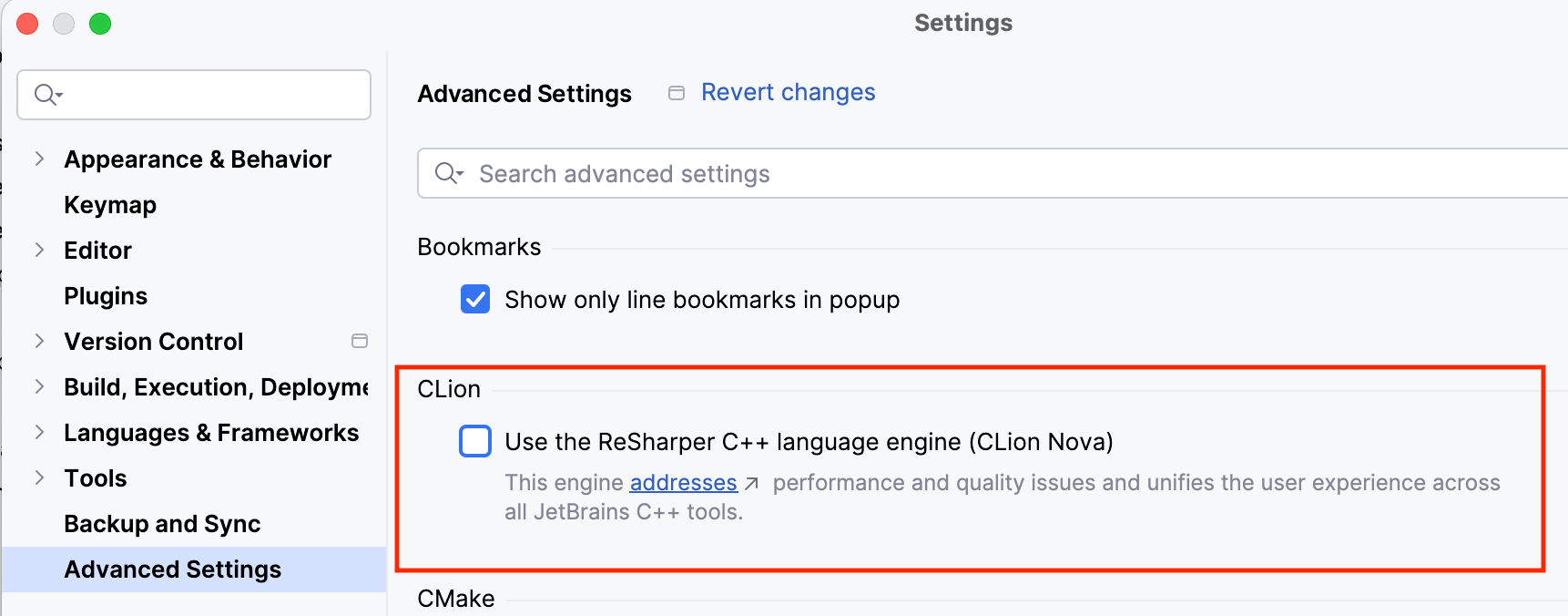
Form Clion 2024.3, Clion Nova is now the default language engine. which use `the ReSharper C++ language engine`. The new language engine is weak of PSI which SequenceDiagram C/C++ is required. So SequenceDiagram C/C++ will report `unsupported language: C++`.
To avoid this, uncheck the `Use the ReSharper C++ language engine(CLion Nova)` Option, in `Settings > Advanced Settings`, under CLion section.
This will roll back to `CLion legacy engine`, which will provide a bunch of PSI to support SequenceDiagram C/C++ to work. The drawback is you lose the advantage of CLion Nova. Your choice.
SequenceDiagram Java
1. Scala for comprehension calls is not supported
For example:
class B() { def bar() = Option("bar") } class A(b: B) { def foo() = { val r = "foo" + b.bar().getOrElse("?") r } def foo2() = { val r = for { x <- b.bar() } yield "foo" + x r.getOrElse("?") } }
the for comprehension call
val r = for { x <- b.bar() } yield "foo" + x
it's UAST tree is `UastEmptyexpression`
UMethod (name = foo2) UBlockExpression UDeclarationsExpression ULocalVariable (name = r) UastEmptyExpression(type = PsiType:Option<String>) UReturnExpression UQualifiedReferenceExpression USimpleNameReferenceExpression (identifier = r) UMethodCall(name = getOrElse) UIdentifier (Identifier (getOrElse)) ULiteralExpression (value = "?")
so the `b.bar()` method call will not generate base on `UastEmptyexpression`. Hopefully, the UAST api will solve this problem sooner.
2. Groovy method body is not supported
For example:
/** * A Class description */ class Student { /** the name of the person */ String name /** * Creates a greeting method for a certain person. * * @param otherPerson the person to greet * @return a greeting message */ //known issue: groovy method will not generate in UAST String greet(String otherPerson) { "Hello ${otherPerson}" // call java Fruit fruit = new Banana() fruit.eat() } }
Based on UAST api limitation, it's UAST tree is no method body mappings.
UFile (package = van.demo.grovvy) UClass (name = Student) UMethod (name = greet) UParameter (name = otherPerson)
When generate sequence of method `greet`, the calls in the method body will not generate (the call java code in the body of `greet`).
"Hello ${otherPerson}" // call java Fruit fruit = new Banana() fruit.eat()